Now the most popular programming languages are JavaScript (25.2 million developers work with it), Python (18.2 million) and Java (17.7 million). But sometimes a project requires specific code that cannot be written using the top popular technologies. Below is a selection of rare programming languages that many developers have never even heard of, but which can give a big boost to development.
COBOL
COBOL (Common Business Oriented Language) appeared in 1959 thanks to Grace Hopper (she is also called the “grandmother of Cobol”). Initially it was imperative and procedural, and since 2002 it became object-oriented. It was disseminated at the initiative of the US Department of Defense and then became widely used in financial computing.
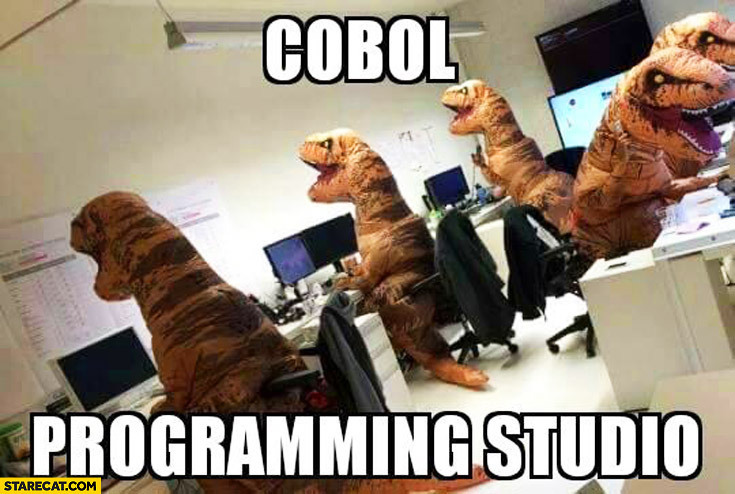
“Using Kobol cripples the mind. Its teaching should therefore be regarded as a criminal offence” – Edsger Dijkstra
To some extent Dijkstra is right – Kobol does have a complex and cumbersome syntax, unlike less “verbose” languages. Nevertheless, it is cool in tasks that other technologies can’t handle. The point is that Kobol performs decimal calculations with fixed point instead of floating point – you don’t need complex rounding rules like, for example, in Java, and operations are faster. Besides, the code will work equally on 8-bit and 64-bit architectures because COBOL can store variables of different types regardless of the compiler and system architecture. That’s why COBOL is indispensable in monetary operations. In the USA, the language is used by banks and the health care system – transactions through terminals, trading on the stock exchange and doctors’ salaries are processed through it.
Here is an example of “simple” code:
IDENTIFICATION DIVISION.
PROGRAM-ID. IDSAMPLE.
ENVIRONMENT DIVISION.
PROCEDURE DIVISION.
DISPLAY 'HELLO WORLD'.
STOP RUN.
No arguments, it doesn’t look as elegant as Python, but all money and sums will be calculated correctly, because rounding of numbers is done not by a processor and arithmetic-logic device, but by a human.
Lua
Lua is a scripting programming language with C source code. It is closest to JavaScript with the same prototypical OOP model, but LUA is more powerful and flexible. Arrays, structures, sets and other composite user-defined data types are implemented using tables, and OOP mechanisms are implemented through meta-tables.
The language was originally developed for industry, rather for the specific oil company Petrobras. Now it is used to develop mass software, for example, for the graphical interface of Adobe Lightroom, and games. Thus, using Lua, you can easily code the behaviour of NPCs (Non-playable characters) and other characters without having to rewrite the engine for them. Among such games are Garry’s Mod, Roblox, Stalker and even World of Warcraft.
Here’s a sample code from Stalker:
local members = {}
local moved = {}
zone = nil
-------------------------------------------------------------------------
function add_member (npc)
--проверим: а наш ли это мужик ?
local ini = npc:spawn_ini ()
if ini == nil or ini:section_exist ("escape_raid") == false then
return
end
--наш, блин. Если уже есть, то пшел вон.
for a = 1, table.getn (members), 1 do
if members[a] == npc.id then
return
end
end
--нету :( Внесем нахала в черный список.
table.insert (members, npc.id)
printf ("Stalker %s is added to list", npc:name ())
-- и изгоним из мира живых
this.switch_offline (npc.id)
end
By the way, you can practice your Lua skills in the Retro Gadgets game. We told you about it in our article.
F#
F Sharp is a multi-paradigm language that supports not only procedural and object-oriented programming, but also functional programming. It was developed in Microsoft Research, and then the company integrated the F# development environment into Visual Studio 2010 and subsequent versions.
F Sharp has concise code with type inference. It is data-oriented, parallel I/O, parallel computation and is often used in scientific research, data analysis, machine learning and web development. Plus, it integrates with .NET platforms.
The language is really rare and unpopular, and there are 100 times fewer specialists who know it than C# developers. However, in some specific tasks related to Big Data and ML, “farshik” can be extremely useful.
Here’s a sample code:
module Integers =
let sampleInteger = 176
/// Do some arithmetic starting with the first integer
let sampleInteger2 = (sampleInteger/4 + 5 - 7) * 4
/// A list of the numbers from 0 to 99
let sampleNumbers = [ 0 .. 99 ]
/// A list of all tuples containing all the numbers from 0 to 99 and their squares
let sampleTableOfSquares = [ for i in 0 .. 99 -> (i, i*i) ]
// The next line prints a list that includes tuples, using %A for generic printing
printfn "The table of squares from 0 to 99 is:\n%A" sampleTableOfSquares
R
Another language for counting numbers and figures – R was created specifically for statistical data analysis. In general, another machine learning and Big Data. It appeared at the University of Auckland to replace the S language, which is paid for and inaccessible to most developers.
Now R is beginning to grow in popularity, and many large companies, such as Amazon, Deloitte, Accenture, Google and others, are using it. Nevertheless, the syntax is not like other languages – it is primarily a statistical technology, so it will be difficult for novice developers to understand it. Among the functions are combining data from tables, creating interactive graphs, and statistical testing. By the way, you can also write a web application in R with the help of the Shiny library.
Here is an example of code that makes a binary code into a decimal code:
# Program to convert decimal
# number into binary number
# using recursive function
convert_to_binary <- function(n) {
if(n > 1) {
convert_to_binary(as.integer(n/2))
}
cat(n %% 2)
}
Clojure
Clojure is a modern, dynamic and functional “dialect” of the Lisp language on the Java platform. It appeared in 2007 thanks to Rich Hickey. Designed as a general purpose language – it combines accessibility and interactive scripting language development with a robust infrastructure for multithreaded programming. Clojure is known for its interactive REPL environment and strong support for parallel programming.
One of the key characteristics of the language is functionality, which means that it emphasises the use of functions and the immutability of data. It also provides access to Java frameworks and can compile with both Java bytecode and JavaScript – useful for both server-side and client-side development.
Clojure is used by big banks like Raiffeisen, as well as Netflix, Amazon, Apple and other giants. For example, Apple uses it to collect data and statistics.
Here, for example, is the code Hello, world on Conjure:
(ns hello
(:gen-class
:methods [[sayHi [] String]]))
(defn -sayHi [this]
(println "hello world"))
Haskell
Another pure functional programming language. Among its features is strict typing, which is why it is named after Haskell Curry, type theory researcher and creator of combinatorial logic. The language also supports deferred calculations.
Haskell is characterised not only by its simple and elegant syntax, but also by its speed and performance. It is used in investment banking, as well as for creating system products and software. Of course, it is also used in large companies: Meta*, IBM, Twitter, Bank of America, and others. For example, Facebook* uses Haskell to fight spam.
Another Hello World, but in Haskell:
main = putStrLn "Hello World!"
Yeah, that’s all.
Elixir
Also a general-purpose programming language that uses dynamic data typing and a functional paradigm, Elixir uses the Erlang virtual machine, known for its ability to run distributed and fault-tolerant systems with low latency.
Features include metaprogramming capabilities that allow developers to write code that writes code, and support for parallel programming. Elixir also provides powerful built-in tools for testing and debugging software.
The language is widely used in web development, especially in the Phoenix Framework, and in the creation of distributed and fault-tolerant systems, thanks to the Erlang/OTP base platform. For developers unfamiliar with functional programming paradigms, it will not be so easy to master, but what advantages – efficiency, scalability and reliability of applications.
Here is the code that will duplicate the strings many times:
"AAA" = String.duplicate("A", 3)
"HELLO HELLO " = String.duplicate("HELLO ", 2)
In fact, there are a lot of niche and rare programming languages. And even experienced developers may have never heard of them. But such languages help to solve specific tasks when popular solutions are powerless.